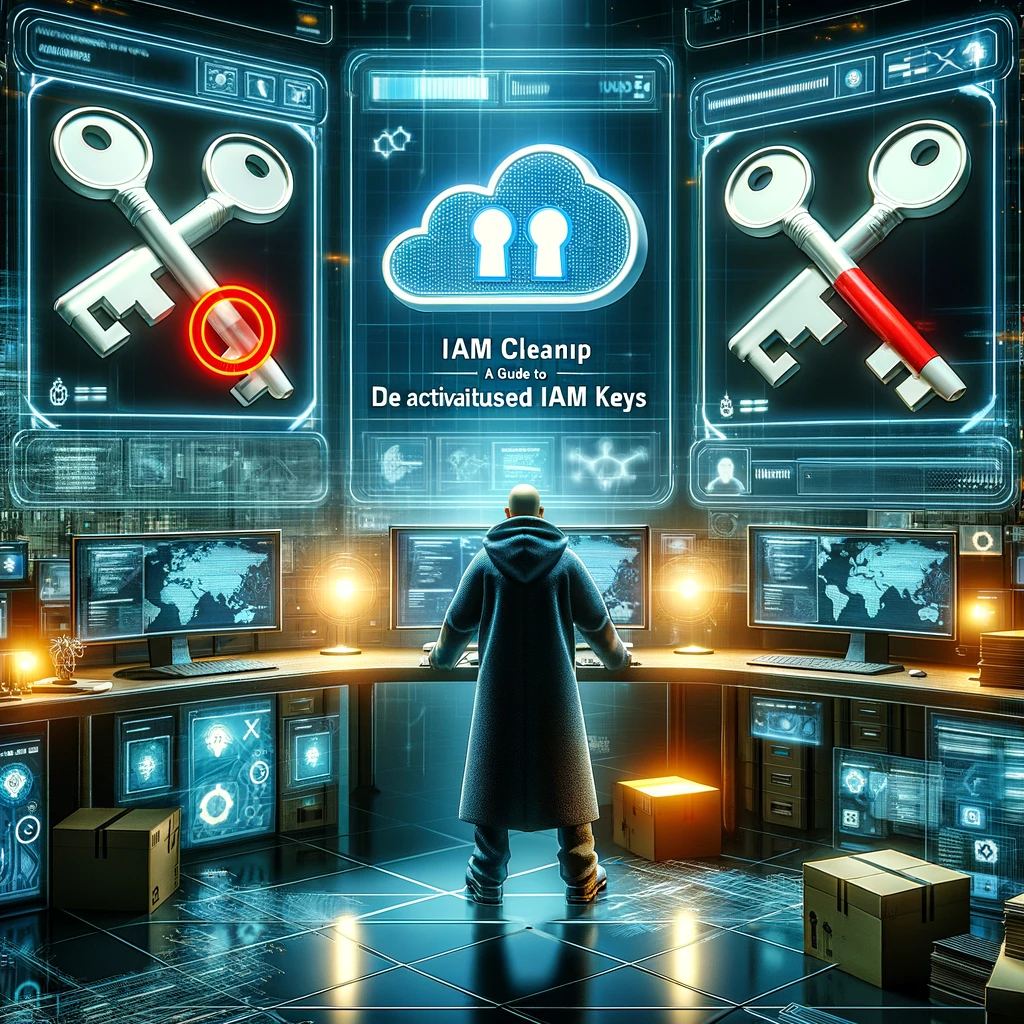
Introduction
Managing access keys for IAM users in AWS is a critical task to ensure the security and compliance of your cloud infrastructure. Access keys provide programmatic access to AWS services and resources, and it’s essential to regularly review and deactivate unused or unnecessary keys. However, manually performing this task can be time-consuming and error-prone, especially in large-scale environments. In this post, we will explore how to automate access key management using a bash script and the AWS CLI. We’ll dive into a practical example that checks the last usage date of access keys for IAM users and deactivates keys that have not been used for 6 months or have never been used.
Welcome to this step-by-step guide on ‘IAM Key Spring Cleaning’. By automating access key management with this script, you can enhance your security posture, reduce manual effort, and ensure that access keys are regularly reviewed and deactivated when necessary.
Use This
- AWS CloudShell
- Bash Script
Do This
- Open CloudShell
- Create bash file: touch deactivateIAMkeys.sh
- Create contents of bash file: vi deactivateIAMkeys.sh
- Type in code below
- Save the file
- Change the file to an executable file: chmod +x deactivateIAMkeys.sh
- Run the script: ./deactivateIAMkeys.sh
Write This
#!/bin/bash
# Get current date
current_date=$(date +%s)
# Calculate date 6 months ago
six_months_ago=$(date -d "6 months ago" +%s)
# Get all users
users=$(aws iam list-users --query "Users[*].UserName" --output text)
# For each user
for user in $users
do
echo "Checking user $user"
# Get their access keys
keys=$(aws iam list-access-keys --user-name $user --query "AccessKeyMetadata[*].AccessKeyId" --output text)
# For each access key
for key in $keys
do
echo "Checking access key $key of user $user"
# Get the last used date for the key
last_used_date=$(aws iam get-access-key-last-used --access-key-id $key | jq -r ".AccessKeyLastUsed.LastUsedDate")
# If last used date is empty, assume the key as not used
if [ "$last_used_date" == "null" ]
then
echo "Key $key of user $user has never been used. Deactivating..."
aws iam update-access-key --user-name $user --access-key-id $key --status Inactive
continue
fi
# Convert last used date to Unix timestamp
last_used_date_unix=$(date -d"$last_used_date" +%s)
# Check if key was used in the last 6 months
if (( last_used_date_unix < six_months_ago ))
then
echo "Key $key of user $user has not been used for 6 months. Deactivating..."
aws iam update-access-key --user-name $user --access-key-id $key --status Inactive
fi
done
done
What The Heck Does This Code Do
- Get today’s date and set up/calculate a variable for a date 6 months ago
- Find all the users in IAM
- Check to see if the user has any access keys
- Get the last date the user used the access key
- If the user never used the access key, deactivate the key
- If the user hasn’t used the access key in the last 6 months, deactivate the key
Till Next Time
Automating access key management in AWS IAM with bash scripting offers numerous benefits in terms of security, efficiency, and compliance. In this post, we explored a practical example of a bash script that utilizes the AWS CLI to check the last usage date of access keys for IAM users and deactivate keys that have not been used for 6 months or have never been used.
By implementing this script, you can streamline the process of access key management, ensuring that inactive or unnecessary keys are promptly deactivated. This helps mitigate the risk of unauthorized access and strengthens the overall security posture of your AWS environment.
Moreover, the script serves as a foundation for customization and extension. You can tailor it to suit your specific requirements, such as sending notifications or integrating it with other processes in your infrastructure.
As your AWS environment evolves, it is crucial to stay proactive in managing access keys and maintaining a strong security stance. Automating access key management with the power of bash scripting and the AWS CLI empowers you to efficiently handle this critical aspect of IAM, allowing you to focus on other essential tasks and ensuring the ongoing integrity and security of your AWS resources.
In the dynamic and often complex world of cloud computing, it’s the small things that can make a big difference. And remember, consistent cloud cleanliness is next to digital godliness. So, keep your digital broom at the ready, sweep away unnecessary objects, and keep your cloud environment running at its peak potential. Until next time, happy ‘Spring Cleaning’!